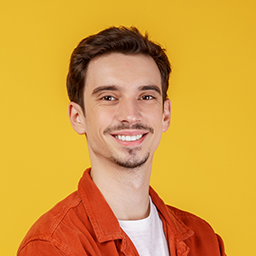
How to create a simple HTML page?
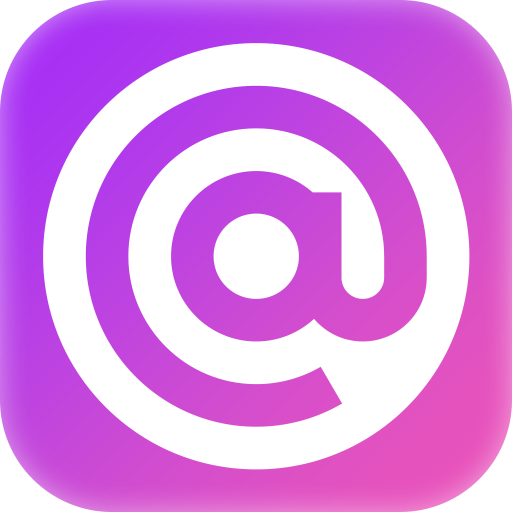
To create a simple HTML page, follow these steps:
-
Open a text editor such as Notepad or Visual Studio Code.
-
Type the following HTML code:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Document</title>
</head>
<body>
<h1>Hello, World!</h1>
<p>This is my first HTML page.</p>
</body>
</html>
-
Save the file with a .html extension, for example,
index.html
. -
Open the saved file in a web browser to view the page.
Explanation:
The code above creates a basic HTML page with a header and body.
<!DOCTYPE html>
specifies the document type as HTML5.
<html>
is the root element and contains all the other elements.
<head>
contains meta information about the document such as the title.
<title>
sets the title of the page, which appears in the browser tab.
<body>
contains the visible content of the page.
<h1>
is a heading element, which is used for the main heading of the page.
<p>
is a paragraph element, which is used for text content.
Save the file with a .html extension and open it in a web browser to see the page.
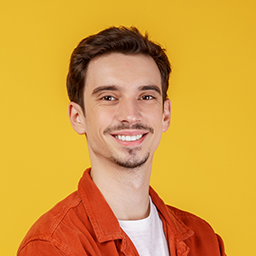
Suggest HTML markup for a block with messages like a Q&A
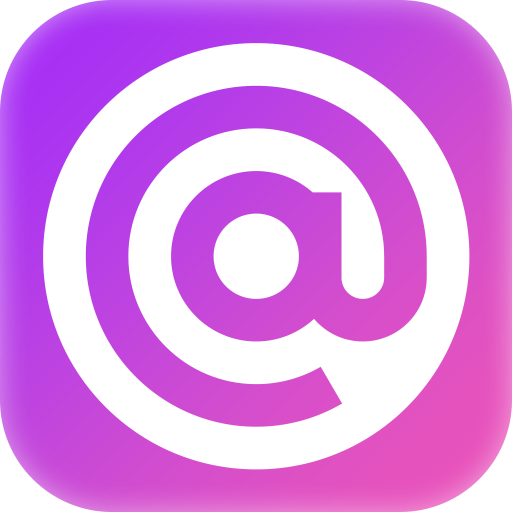
To create a block with messages like a Q&A, you can use HTML markup such as a list with nested elements. Here's an example:
<ul>
<li>
<strong>Question:</strong> What is HTML?
<ul>
<li><strong>Answer:</strong> HTML stands for Hypertext Markup Language, which is used to create web pages.</li>
</ul>
</li>
<li>
<strong>Question:</strong> What are some common HTML tags?
<ul>
<li><strong>Answer:</strong> Some common HTML tags include <html>, <head>, <body>, <p>, and <a>.</li>
</ul>
</li>
<li>
<strong>Question:</strong> How do you add an image to a web page?
<ul>
<li><strong>Answer:</strong> To add an image, use the <img> tag and specify the image source using the "src" attribute.</li>
</ul>
</li>
</ul>
Explanation:
- The
<ul>
element creates an unordered list. - Each question and answer pair is represented by an
<li>
element. - The question is displayed using the
<strong>
element to make it bold. - The answer is displayed within a nested unordered list using the
<ul>
and<li>
elements. - The answer is displayed using the
<strong>
element to make it bold. - Code snippets are displayed using the
<code>
element.
You can modify this markup to suit your needs, such as adding more questions and answers, changing the formatting, or using different HTML elements.
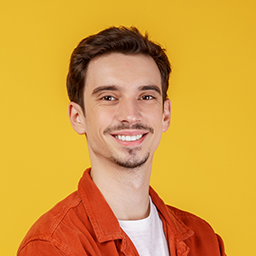
Provide HTML markup with complex class names for Q&A message block
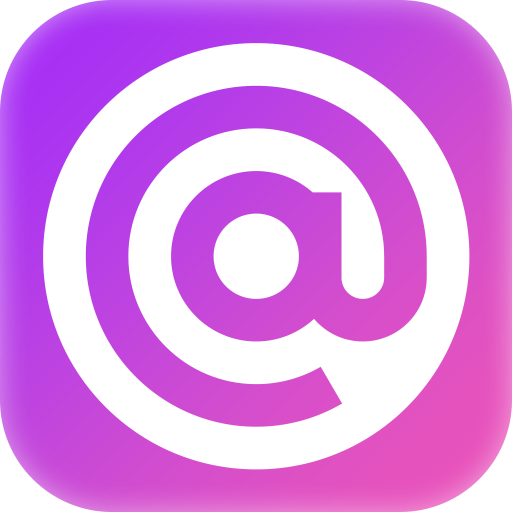
Here's an example of HTML markup with complex class names for a Q&A message block:
<div class="message-block">
<div class="message">
<div class="message-question">
<p class="question-text">What is HTML?</p>
<i class="question-icon fas fa-question-circle"></i>
</div>
<div class="message-answer">
<p class="answer-text">HTML stands for Hypertext Markup Language, which is used to create web pages.</p>
<i class="answer-icon fas fa-check-circle"></i>
</div>
</div>
<div class="message">
<div class="message-question">
<p class="question-text">What are some common HTML tags?</p>
<i class="question-icon fas fa-question-circle"></i>
</div>
<div class="message-answer">
<p class="answer-text">Some common HTML tags include <html>, <head>, <body>, <p>, and <a>.</p>
<i class="answer-icon fas fa-check-circle"></i>
</div>
</div>
<div class="message">
<div class="message-question">
<p class="question-text">How do you add an image to a web page?</p>
<i class="question-icon fas fa-question-circle"></i>
</div>
<div class="message-answer">
<p class="answer-text">To add an image, use the <img> tag and specify the image source using the "src" attribute.</p>
<i class="answer-icon fas fa-check-circle"></i>
</div>
</div>
</div>
Explanation:
- The outermost
div
element has a class ofmessage-block
to group all the Q&A messages together. - Each Q&A message is contained within a
div
element with a class ofmessage
. - The question and answer are each contained within a
div
element with a class ofmessage-question
andmessage-answer
, respectively. - The text of the question and answer are contained within
p
elements with classes ofquestion-text
andanswer-text
, respectively. - Each question and answer is accompanied by an icon, represented by an
i
element with a fontawesome class offas fa-question-circle
for the question icon, andfas fa-check-circle
for the answer icon. - Each icon has a class of
question-icon
oranswer-icon
, respectively.
You can modify these class names to suit your needs and apply custom styles to the elements using CSS.